LeetCode 1207 solution
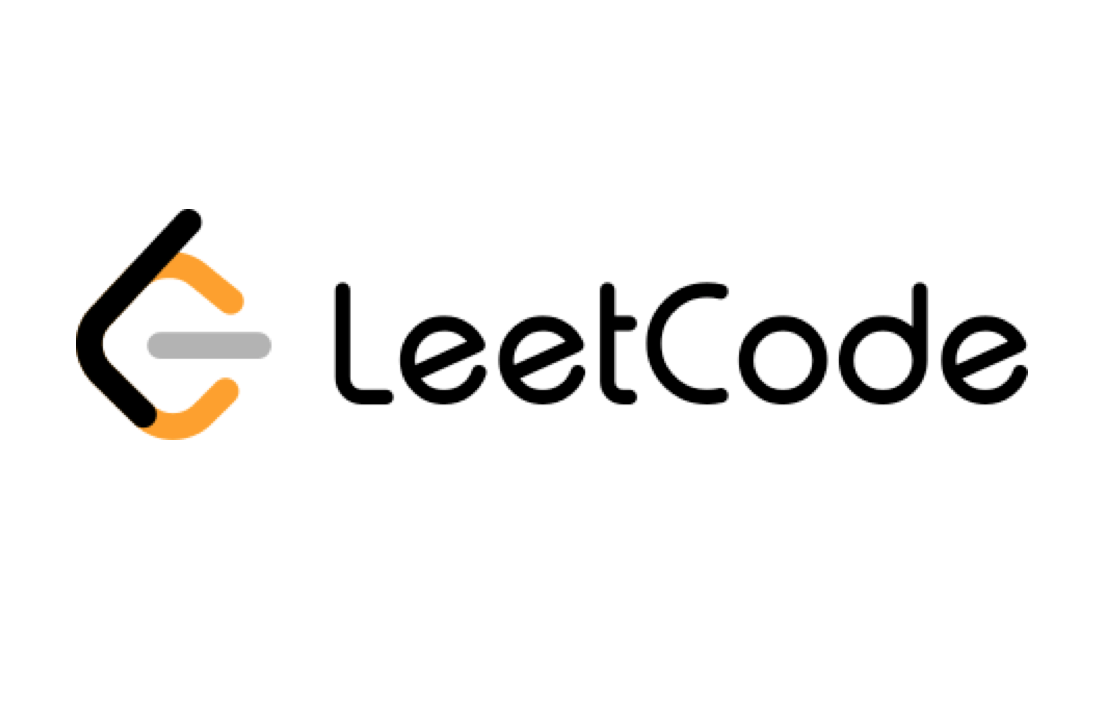
problem
Given an array of integersarr
, returntrue
if the number of occurrences of each value in the array is unique orfalse
otherwise.
Example 1:
- Input: arr = [1,2,2,1,1,3]
- Output: true
- Explanation: The value 1 has 3 occurrences, 2 has 2 and 3 has 1. No two values have the same number of occurrences.
python
class Solution:
def uniqueOccurrences(self, arr: List[int]) -> bool:
# hash table
hash_t = {}
for i in arr:
if i in hash_t:
hash_t[i] += 1
else:
hash_t[i] = 1
hash_t_ = {}
for j in hash_t.values():
if j in hash_t_:
hash_t_[j] += 1
else:
hash_t_[j] = 1
for k in hash_t_.values():
if k > 1:
return False
return True
The first thought that come up with my mind is that "I should use hash table with this problem". Occurrences of each number needs to be checked. And also, the recorded hash tables needs to be checked one more time with hash table. With this two step, I could solve the problem.
The best thing about hash table is that it is Fast data retrieval structure. At very most time for algorithm problem, using hash table for data search and store is faster than just search with for loop. Because of the "key-value pairs and hash function", this advantage($O(1)$) is possible.
other solution
This is solution from MarkSPhilips31
class Solution:
def uniqueOccurrences(self, arr: List[int]) -> bool:
freq = {}
for x in arr:
freq[x] = freq.get(x, 0) + 1
return len(freq) == len(set(freq.values()))
The code is so short... and clean. Making hash table is same as me, but to check whether there is over 1 occurrence happen, the len(freq) == len(set(freq.values()))
part is soooo dope. Eduacative