242. Valid Anagram

Given two stringss
andt
, returntrue
ift
is an anagram ofs
, andfalse
otherwise.
An Anagram is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.

First solv

class Solution:
def isAnagram(self, s: str, t: str) -> bool:
def checker(x:str):
check = {}
for i in x:
if i not in check:
check[i] = 1
else:
check[i] += 1
return check
check_s, check_t = checker(s), checker(t)
if check_s == check_t:
return True
else:
return False
It is easy to solve this problem with hash table
. Cause need to make hash table exactly same way for s
and t
, made a checker
function. It looks like this {"a":1, "b":2}
. The dict which is hash table in python has these features.
- key never duplicated
- it is set with value. So no need to be sorted
So with these features, just make 2 dictionary and compared it.
ring my bell
My solution has middle level of runtime and memory efficiency. One solution I saw and ringed my bell is this solution.
class Solution:
def isAnagram(self, s: str, t: str) -> bool:
s_count = Counter(s)
t_count = Counter(t)
return s_count == t_count
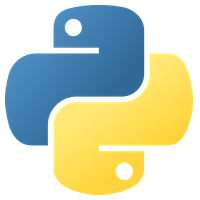
The Counter in python in built in class for a dict
subclass for counting hashable objects. It is exactly what we want for this problem and it is always good to use built in solution in python.